mirror of
https://github.com/donnemartin/data-science-ipython-notebooks.git
synced 2024-03-22 13:30:56 +08:00
Added slice snippets
This commit is contained in:
parent
ff31684ee5
commit
60b1901186
|
@ -1,7 +1,7 @@
|
|||
{
|
||||
"metadata": {
|
||||
"name": "",
|
||||
"signature": "sha256:d7e86a84d9ac272fcd99e1aadbc8c73b80d1affd3c0533649f6842229dee5f2b"
|
||||
"signature": "sha256:f24f6112f8e8e28d262a4f84be84d1ae459154a9a445687d55392ff177e6a03c"
|
||||
},
|
||||
"nbformat": 3,
|
||||
"nbformat_minor": 0,
|
||||
|
@ -574,8 +574,8 @@
|
|||
"\n",
|
||||
"# Find the location where an element should be inserted to keep the\n",
|
||||
"# list sorted\n",
|
||||
"c_list = [1, 2, 2, 4, 8, 10]\n",
|
||||
"bisect.bisect(c_list, 5)"
|
||||
"c_list = [1, 2, 2, 3, 5, 13]\n",
|
||||
"bisect.bisect(c_list, 8)"
|
||||
],
|
||||
"language": "python",
|
||||
"metadata": {},
|
||||
|
@ -583,20 +583,20 @@
|
|||
{
|
||||
"metadata": {},
|
||||
"output_type": "pyout",
|
||||
"prompt_number": 40,
|
||||
"prompt_number": 25,
|
||||
"text": [
|
||||
"4"
|
||||
"5"
|
||||
]
|
||||
}
|
||||
],
|
||||
"prompt_number": 40
|
||||
"prompt_number": 25
|
||||
},
|
||||
{
|
||||
"cell_type": "code",
|
||||
"collapsed": false,
|
||||
"input": [
|
||||
"# Inserts an element into a location to keep the list sorted\n",
|
||||
"bisect.insort(c_list, 5)\n",
|
||||
"bisect.insort(c_list, 8)\n",
|
||||
"c_list"
|
||||
],
|
||||
"language": "python",
|
||||
|
@ -605,13 +605,204 @@
|
|||
{
|
||||
"metadata": {},
|
||||
"output_type": "pyout",
|
||||
"prompt_number": 41,
|
||||
"prompt_number": 26,
|
||||
"text": [
|
||||
"[1, 2, 2, 4, 5, 8, 10]"
|
||||
"[1, 2, 2, 3, 5, 8, 13]"
|
||||
]
|
||||
}
|
||||
],
|
||||
"prompt_number": 41
|
||||
"prompt_number": 26
|
||||
},
|
||||
{
|
||||
"cell_type": "markdown",
|
||||
"metadata": {},
|
||||
"source": [
|
||||
"## slice"
|
||||
]
|
||||
},
|
||||
{
|
||||
"cell_type": "markdown",
|
||||
"metadata": {},
|
||||
"source": [
|
||||
"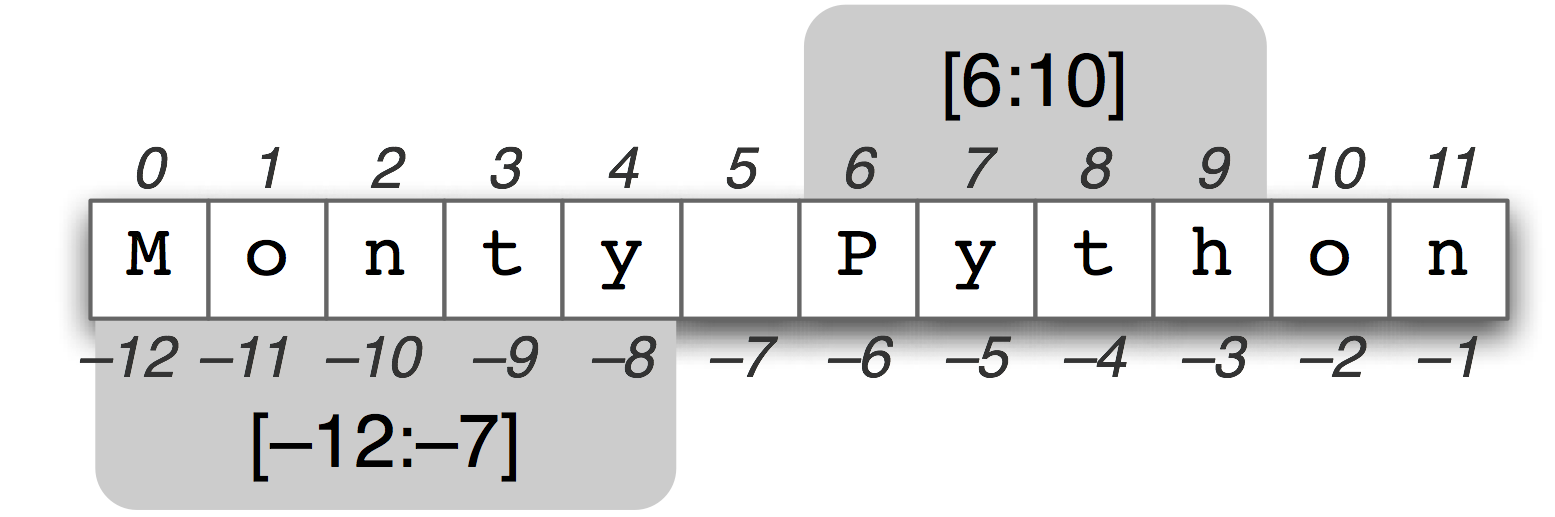"
|
||||
]
|
||||
},
|
||||
{
|
||||
"cell_type": "code",
|
||||
"collapsed": false,
|
||||
"input": [
|
||||
"# Select a section of list types (arrays, tuples, NumPy arrays)\n",
|
||||
"# start:stop\n",
|
||||
"# start is included, stop is not\n",
|
||||
"# number of elements in the result is stop - start\n",
|
||||
"d_list = 'Monty Python'\n",
|
||||
"d_list[6:10]"
|
||||
],
|
||||
"language": "python",
|
||||
"metadata": {},
|
||||
"outputs": [
|
||||
{
|
||||
"metadata": {},
|
||||
"output_type": "pyout",
|
||||
"prompt_number": 27,
|
||||
"text": [
|
||||
"'Pyth'"
|
||||
]
|
||||
}
|
||||
],
|
||||
"prompt_number": 27
|
||||
},
|
||||
{
|
||||
"cell_type": "code",
|
||||
"collapsed": false,
|
||||
"input": [
|
||||
"# Omit start to default to start of the sequence\n",
|
||||
"d_list[:5]"
|
||||
],
|
||||
"language": "python",
|
||||
"metadata": {},
|
||||
"outputs": [
|
||||
{
|
||||
"metadata": {},
|
||||
"output_type": "pyout",
|
||||
"prompt_number": 28,
|
||||
"text": [
|
||||
"'Monty'"
|
||||
]
|
||||
}
|
||||
],
|
||||
"prompt_number": 28
|
||||
},
|
||||
{
|
||||
"cell_type": "code",
|
||||
"collapsed": false,
|
||||
"input": [
|
||||
"# Omit end to default to end of the sequence\n",
|
||||
"d_list[6:]"
|
||||
],
|
||||
"language": "python",
|
||||
"metadata": {},
|
||||
"outputs": [
|
||||
{
|
||||
"metadata": {},
|
||||
"output_type": "pyout",
|
||||
"prompt_number": 29,
|
||||
"text": [
|
||||
"'Python'"
|
||||
]
|
||||
}
|
||||
],
|
||||
"prompt_number": 29
|
||||
},
|
||||
{
|
||||
"cell_type": "code",
|
||||
"collapsed": false,
|
||||
"input": [
|
||||
"# Negative indices slice relative to the end\n",
|
||||
"d_list[-12:-7]"
|
||||
],
|
||||
"language": "python",
|
||||
"metadata": {},
|
||||
"outputs": [
|
||||
{
|
||||
"metadata": {},
|
||||
"output_type": "pyout",
|
||||
"prompt_number": 30,
|
||||
"text": [
|
||||
"'Monty'"
|
||||
]
|
||||
}
|
||||
],
|
||||
"prompt_number": 30
|
||||
},
|
||||
{
|
||||
"cell_type": "code",
|
||||
"collapsed": false,
|
||||
"input": [
|
||||
"# Slice can also take a step such as the one below, which takes\n",
|
||||
"# every other element\n",
|
||||
"e_list[::2]"
|
||||
],
|
||||
"language": "python",
|
||||
"metadata": {},
|
||||
"outputs": [
|
||||
{
|
||||
"metadata": {},
|
||||
"output_type": "pyout",
|
||||
"prompt_number": 33,
|
||||
"text": [
|
||||
"[1, 2, 5, 13]"
|
||||
]
|
||||
}
|
||||
],
|
||||
"prompt_number": 33
|
||||
},
|
||||
{
|
||||
"cell_type": "code",
|
||||
"collapsed": false,
|
||||
"input": [
|
||||
"# Passing -1 for the step reverses the list or tuple:\n",
|
||||
"e_list[::-1]"
|
||||
],
|
||||
"language": "python",
|
||||
"metadata": {},
|
||||
"outputs": [
|
||||
{
|
||||
"metadata": {},
|
||||
"output_type": "pyout",
|
||||
"prompt_number": 34,
|
||||
"text": [
|
||||
"[13, ['H', 'a', 'l', 'l'], 5, 3, 2, 1, 1]"
|
||||
]
|
||||
}
|
||||
],
|
||||
"prompt_number": 34
|
||||
},
|
||||
{
|
||||
"cell_type": "code",
|
||||
"collapsed": false,
|
||||
"input": [
|
||||
"# Assign elements to a slice\n",
|
||||
"# Slice range does not have to equal number of elements to assign\n",
|
||||
"e_list = [1, 1, 2, 3, 5, 8, 13]\n",
|
||||
"e_list[5:] = ['H', 'a', 'l', 'l']\n",
|
||||
"e_list"
|
||||
],
|
||||
"language": "python",
|
||||
"metadata": {},
|
||||
"outputs": [
|
||||
{
|
||||
"metadata": {},
|
||||
"output_type": "pyout",
|
||||
"prompt_number": 31,
|
||||
"text": [
|
||||
"[1, 1, 2, 3, 5, 'H', 'a', 'l', 'l']"
|
||||
]
|
||||
}
|
||||
],
|
||||
"prompt_number": 31
|
||||
},
|
||||
{
|
||||
"cell_type": "code",
|
||||
"collapsed": false,
|
||||
"input": [
|
||||
"# Compare assigning into a slice (above) versus assigning into\n",
|
||||
"# an inde\n",
|
||||
"e_list"
|
||||
],
|
||||
"language": "python",
|
||||
"metadata": {},
|
||||
"outputs": [
|
||||
{
|
||||
"metadata": {},
|
||||
"output_type": "pyout",
|
||||
"prompt_number": 32,
|
||||
"text": [
|
||||
"[1, 1, 2, 3, 5, ['H', 'a', 'l', 'l'], 13]"
|
||||
]
|
||||
}
|
||||
],
|
||||
"prompt_number": 32
|
||||
},
|
||||
{
|
||||
"cell_type": "code",
|
||||
|
|
Loading…
Reference in New Issue
Block a user